C program to merge two arrays into third array: Arrays are assumed to be sorted in ascending order. You enter two short sorted arrays and combine them to get a large array.
Download Merge arrays program.
Output of program:
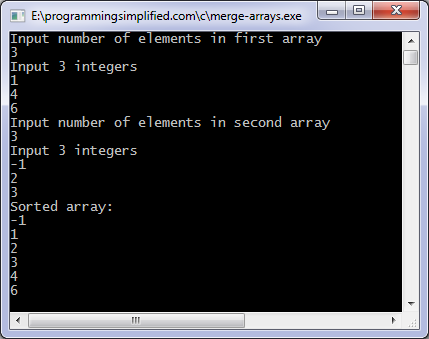
If the arrays are not sorted then you can sort them first and then use the above merge function, another method is to merge them and then sort the array. Sorting two smaller arrays will take less time as compared to sorting a big array. Merging two sorted array is used in merge sort algorithm.
C programming code to merge two sorted arrays
#include <stdio.h> void merge(int [], int, int [], int, int []); int main() { int a[100], b[100], m, n, c, sorted[200]; printf("Input number of elements in first array\n"); scanf("%d", &m); printf("Input %d integers\n", m); for (c = 0; c < m; c++) { scanf("%d", &a[c]); } printf("Input number of elements in second array\n"); scanf("%d", &n); printf("Input %d integers\n", n); for (c = 0; c < n; c++) { scanf("%d", &b[c]); } merge(a, m, b, n, sorted); printf("Sorted array:\n"); for (c = 0; c < m + n; c++) { printf("%d\n", sorted[c]); } return 0; } void merge(int a[], int m, int b[], int n, int sorted[]) { int i, j, k; j = k = 0; for (i = 0; i < m + n;) { if (j < m && k < n) { if (a[j] < b[k]) { sorted[i] = a[j]; j++; } else { sorted[i] = b[k]; k++; } i++; } else if (j == m) { for (; i < m + n;) { sorted[i] = b[k]; k++; i++; } } else { for (; i < m + n;) { sorted[i] = a[j]; j++; i++; } } } }
Output of program:
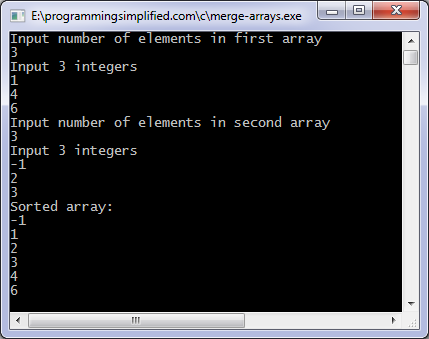
If the arrays are not sorted then you can sort them first and then use the above merge function, another method is to merge them and then sort the array. Sorting two smaller arrays will take less time as compared to sorting a big array. Merging two sorted array is used in merge sort algorithm.
No comments:
Post a Comment